To pass data between different browsing contexts like passing data between 2 open tabs, passing data between a tab and service worker, or any context of the same origin in JavaScript, you can use the BroadcastChannel
API.
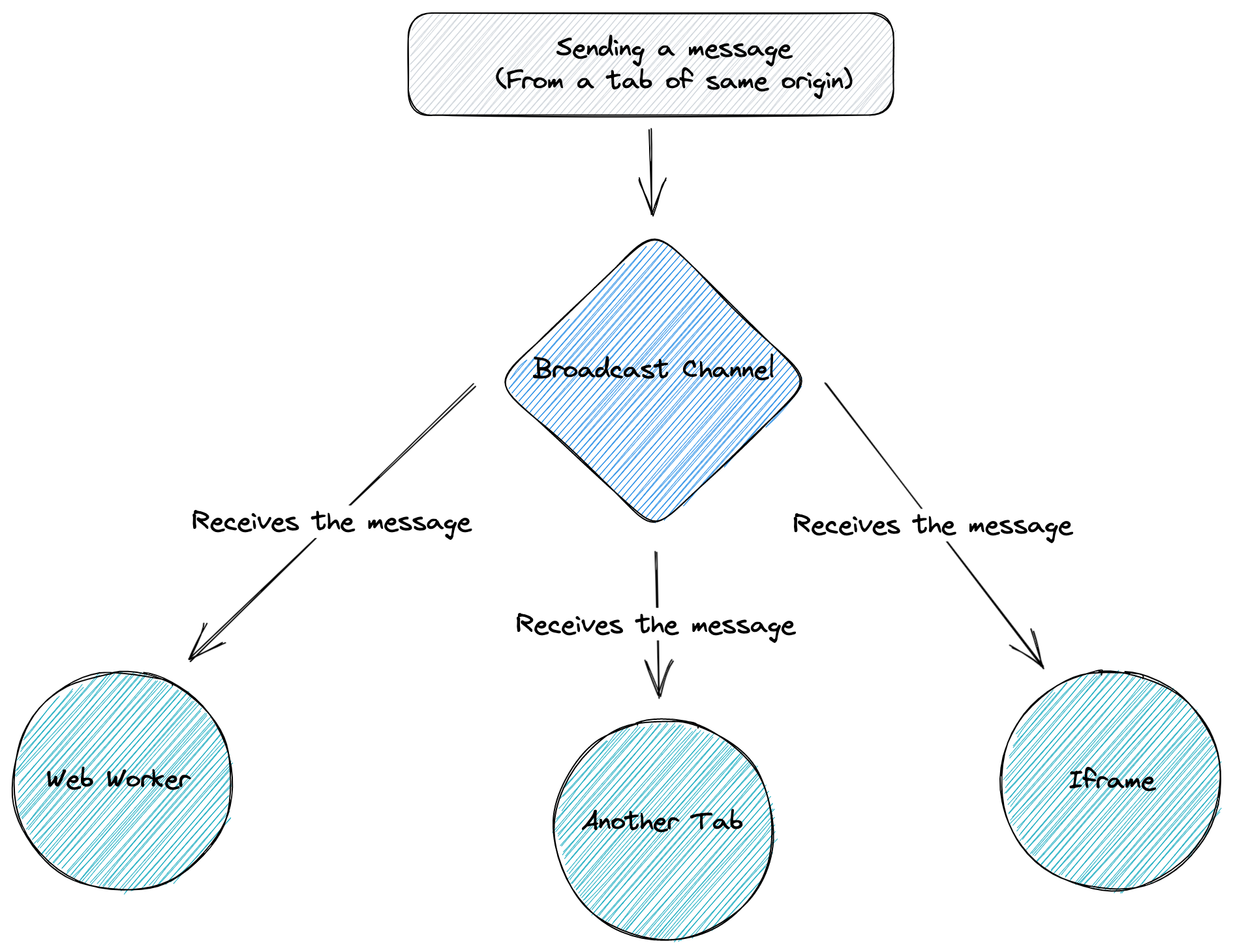
Broadcast Channel Web API Demo
Steps to see the demo :
- First, Open a new tab with the same page. You can also click here to open a new tab with the same webpage quickly.
- Secondly, Type your message on the above input and click on the
Broadcast Message
button. - Thirdly, Now to go the another tab you just opened, you can see that an
alert
popup is shown with your message. This is done using theBroadcast Channel Web API
.
Usage
First, you have to make an instance of BroadcastChannel
with the channel name of your choice.
The channel name should be passed as an argument to the BroadcastChannel()
as a string.
// create an instance of BroadcastChannel
// with channel name as test_channel
const channel = new BroadcastChannel("test_channel");
After creating an instance you need to add a message
event listener to the channel
object so that when you receive messages the callback function inside the event handler is called.
// create an instance of BroadcastChannel
const channel = new BroadcastChannel("test_channel");
// add message event listener to receive meesage
channel.addEventListener("message", (event) => {
// data property
console.log(event.data);
});
The callback function is passed with an event
object.
You can find the data inside the data
property inside the event object.
Now you may think how will I send data?
For that you have to use the postMessage()
method in the channel
object.
// create an instance of BroadcastChannel
const channel = new BroadcastChannel("test_channel");
// add message event listener to receive meesage
channel.addEventListener("message", (event) => {
// data
console.log(event.data);
});
// send some data or message
channel.postMessage({
name: "John Doe",
age: 23,
});
Now to test this open a new tab of the website and when you open you can see that the data { name: "John Doe", age: 23 }
is shown in the console.
For testing, you can head on to this JS Bin and open a new tab of the same JS BIN URL to see the result.