To write a pattern similar to the while loop in Go or Golang, you have to use the for
keyword followed by the condition to run the loop and then the opening and closing curly brackets symbol ({}
). There is no while
keyword to do the while looping in Go like in other programming languages you may be familiar with. The for
keyword is used to do the while looping in Go.
TL;DR
package main
import "fmt"
func main(){
// variable to hold user answer
var userAnswer string
// check if the `userAnswer` variable has the
// value that is not equal to string `magic`
// using the `for` loop.
// The `for` loop will keep on looping
// until the condition becomes `false`
// this is similar to the working of a `while` loop
for userAnswer != "magic"{
// show the prompt to the user
fmt.Println("Please enter the 'magic' keyword.")
// read the value from the terminal
fmt.Scanln(&userAnswer)
}
// show output when user enters the correct word
fmt.Println("You Won The Game!")
}
For example, let's say we need to create a game program where we ask the users to enter a magic keyword, if the user enters the word magic
then we have to show to the user that he has won the game else we have to keep on asking the user to enter the word.
To do this, we need a for
loop where we have to keep on asking the question Please enter the 'magic' keyword.
First, let's declare a variable to hold the user's answer.
It can be done like this,
package main
func main(){
// variable to hold user answer
var userAnswer string
}
Now let's write the for
loop where we will write the condition to check if the userAnswer
variable value is not equal to the string
value of magic
. Only if the user's answer is not the string magic
then we need to loop to show the question.
It can be done like this,
package main
func main(){
// variable to hold user answer
var userAnswer string
// check if the `userAnswer` variable has the
// value that is not equal to string `magic`
// using the `for` loop.
// The `for` loop will keep on looping
// until the condition becomes `false`
// this is similar to the working of a `while` loop
for userAnswer != "magic"{
// cool code here
}
}
Now we need to show the user a prompt in the terminal like Please enter the 'magic' keyword.
and then accept a value from the terminal and save that value to the userAnswer
variable. To do that we can use the Scanln()
method from the fmt
standard package and then pass the variable address to it as its argument. The Scanln()
method helps us to read values from the terminal.
It can be done like this,
package main
import "fmt"
func main(){
// variable to hold user answer
var userAnswer string
// check if the `userAnswer` variable has the
// value that is not equal to string `magic`
// using the `for` loop.
// The `for` loop will keep on looping
// until the condition becomes `false`
// this is similar to the working of a `while` loop
for userAnswer != "magic"{
// show the prompt to the user
fmt.Println("Please enter the 'magic' keyword.")
// read the value from the terminal
fmt.Scanln(&userAnswer)
}
}
Finally, we need to show the string
of You Won The Game!
once the user enters the correct word.
It can be done like this,
package main
import "fmt"
func main(){
// variable to hold user answer
var userAnswer string
// check if the `userAnswer` variable has the
// value that is not equal to string `magic`
// using the `for` loop.
// The `for` loop will keep on looping
// until the condition becomes `false`
// this is similar to the working of a `while` loop
for userAnswer != "magic"{
// show the prompt to the user
fmt.Println("Please enter the 'magic' keyword.")
// read the value from the terminal
fmt.Scanln(&userAnswer)
}
// show output when user enters the correct word
fmt.Println("You Won The Game!")
}
If you run the above code, you can see that a prompt will appear for you to write the word and if you enter any other string other than the word magic it will keep on showing the prompt of Please enter the 'magic' keyword.
which is due to the for
loop condition not being satisfied.
It may look like this,
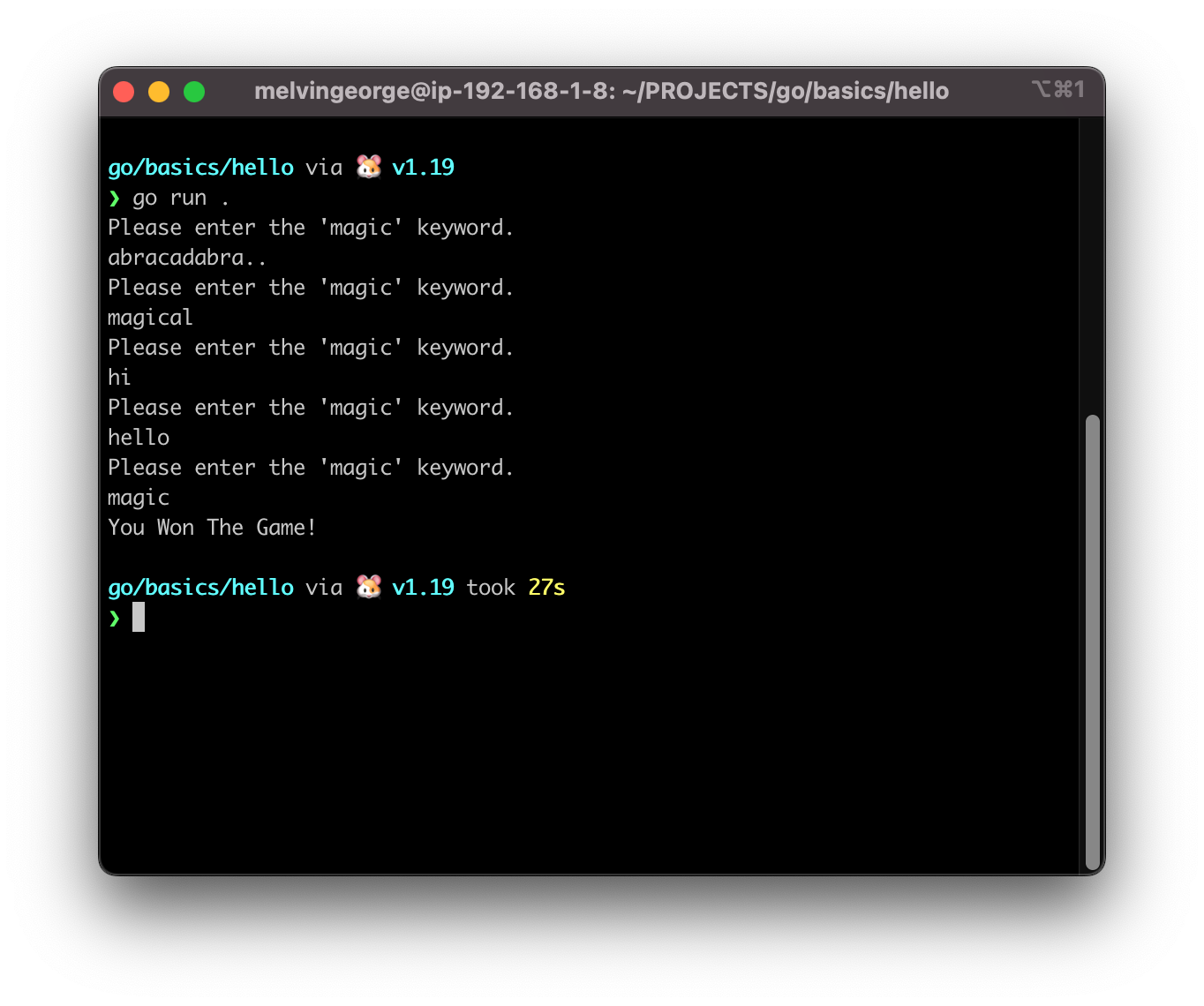
We have successfully written a while loop analogous to using for loop in Go. Yay 🥳!
That's all 😃.