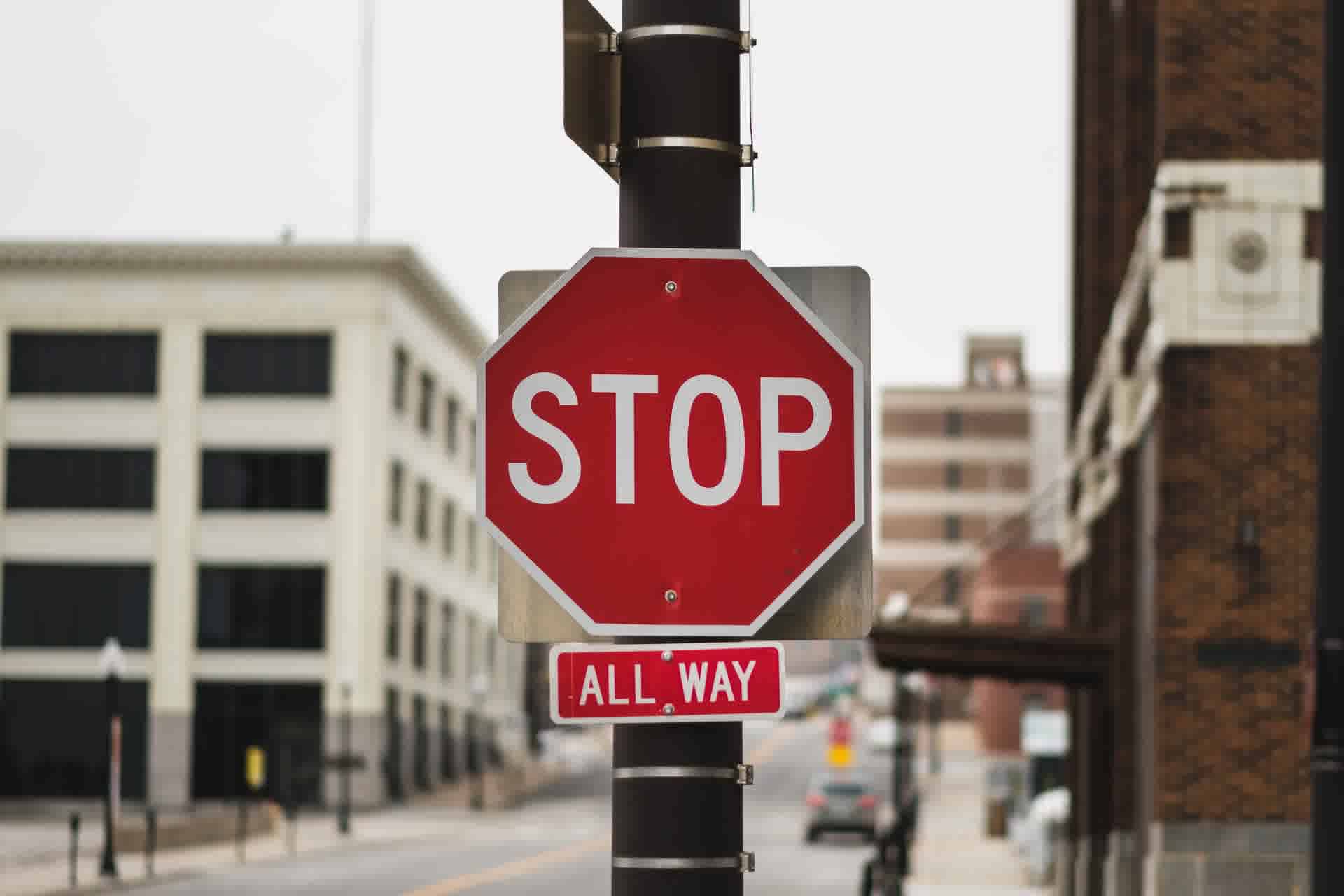
Photo by
John Matychuk
Whenever we program an application we need logging of information to know what is happening with the application. It may be for error detection or for simple information logging.
So logging is an essential part of an application and different situations need different types of logging.
Let's discuss some different types of logging available in JavaScript.
- General logging using
console.log()
andconsole.debug()
methods - Examining contents of object using
console.dir()
method - Logging error and warnings with
console.error()
andconsole.warn()
methods - Examining content as tabular data using
console.table()
method
General logging using console.log()
and console.debug()
methods
We generally log information in JavaScript using the console.log()
method.
console.log("Hello World!"); // Hello World!
We can pass many log messages as arguments to the console.log()
method.
console.log("Hello World!", "How are you?"); // Hello World! How are you?
We can also use the console.log()
method to examing contents of an object.
const Earth = {
name: "Earth",
age: "A Trillion Years",
};
// log object Earth
console.log(Earth); // {name: "Earth", age: "A Trillion Years"}
We can also log information using the console.debug()
method. This is just like the console.log()
method but instead, the information logged using this can be seen only if the console is in debugging level.
Examining contents of object using console.dir()
method
While you can also examine the contents of objects using the console.log()
method, this is no different from the console.dir()
method which can also be used to examine the contents of an object.
But there is a slight difference to it.
If you are trying to view the contents of an HTML element using the console.log()
method, you would see the content int he console just like how it is represented in plain HTML, not useful if you are trying to view the attributes and all other cool kinds of stuff.
<div id="section">
<h1>Hello World!</h1>
</div>
// get reference to div tag
const section = document.getElementById("section");
// log contents of div tag using console.log() method
console.log(section);
/*
RESULT:
=======
<div id="section">
<h1>Hello World!</h1>
</div>
=======
*/
But if you try to view this using the console.dir()
method, you could see all the attributes and other cool stuff for the div
tag.
// get reference to div tag
const section = document.getElementById("section");
// log contents of div tag using console.dir() method
console.dir(section);
/*
RESULT:
=======
accessKey: ""
align: ""
ariaAtomic: null
ariaAutoComplete: null
ariaBusy: null
ariaChecked: null
ariaColCount: null
.
.
.
. etc
=======
*/
Logging error and warnings with console.error()
and console.warn()
methods
If you want to log an error instead of some information you can use the console.error()
method.
// error
console.error("This is a big error 🚫");

The error will be in red color and will also show the line number where the error has happened.
Or if you want to log a warning instead of a pure error, you can use the console.warn()
method.
// warning
console.warn("This is just a warning ⚠️");

Examining content as tabular data using console.table()
method
If you want to view your contents of objects in a table-like structure, you can use the console.table()
method.
// object
const John = {
name: "John Doe",
age: 23,
};
// examine contents in a table like structure
// using console.table() method
console.table(John);
