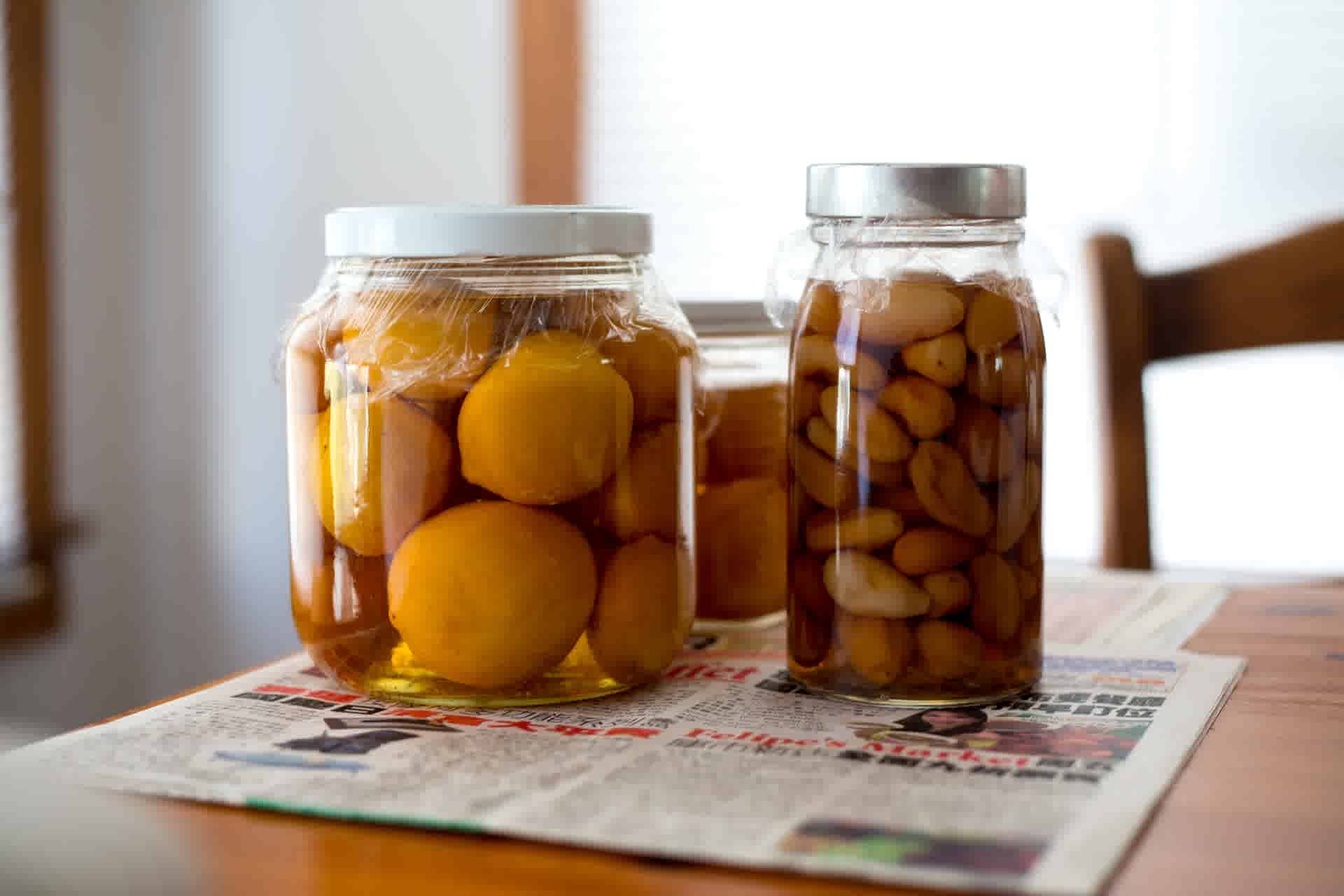
Photo by
Jason Leung
When you build a web application, often we might need to save data for a user across visits to the website in the future.
You can achieve this functionality with the following:-
localStorage
Browser API: for storing data with no expiration datesessionStorage
Browser API: for storing data for just one session i.e, data will be lost when the browser tab is closed
Local Storage
localStorage
is a Web API for storing data without any expiration date. The data wouldn't be deleted when the browser tab is closed, and hence would be available the next day, week, month, or year.
. We can store data into it in a key/pair format.
Storing Data
To store data we need to use the setItem()
method available in the localStorage
object.
Syntax
localStorage.setItem("key", "value");
Let's store our user's names.
const name = "John Doe";
// storing user's name
localStorage.setItem("name", name);
The method accepts:
- the name of the key as the first argument
- the value to store as the second argument
Get Stored Data
To get the stored data we need to use the getItem()
method available in the localStorage
object.
Syntax
localStorage.getItem("key");
Let's retrieve the stored user's name
// get stored name
const name = localStorage.getItem("name");
The method accepts:
- the name of the key as an argument.
- the method returns the stored value for the key name supplied
Removing Stored Data
To remove stored data we need to use the removeItem()
method available in the localStorage
object.
Syntax
localStorage.removeItem("key");
Let's remove the stored user's name
// remove the stored user's name
localStorage.removeItem("name");
The method accepts:
- the key name to remove as an argument.
For clearing all saved data from local storage use localStorage.clear();
Session Storage
The sessionStorage object is similar to the localStorage object, except that it stores the data for only one session and this data will be cleared when the page session ends. Thus the sessionStorage exists only within the current browser tab and another tab with the same page will have different storage.
Storing Data
To store data we need to use the setItem()
method available in the sessionStorage
object.
Let's store our user's names.
// storing user's name
sessionStorage.setItem("name", "Tom");
Get Stored Data
To get the stored data we need to use the getItem()
method available in the sessionStorage
object.
Let's retrieve the stored user's name
// get stored name
const name = sessionStorage.getItem("name");
Removing Stored Data
To remove stored data we need to use the removeItem()
method available in the sessionStorage
object.
Let's remove the stored user's name
// remove the stored user's name
sessionStorage.removeItem("name");