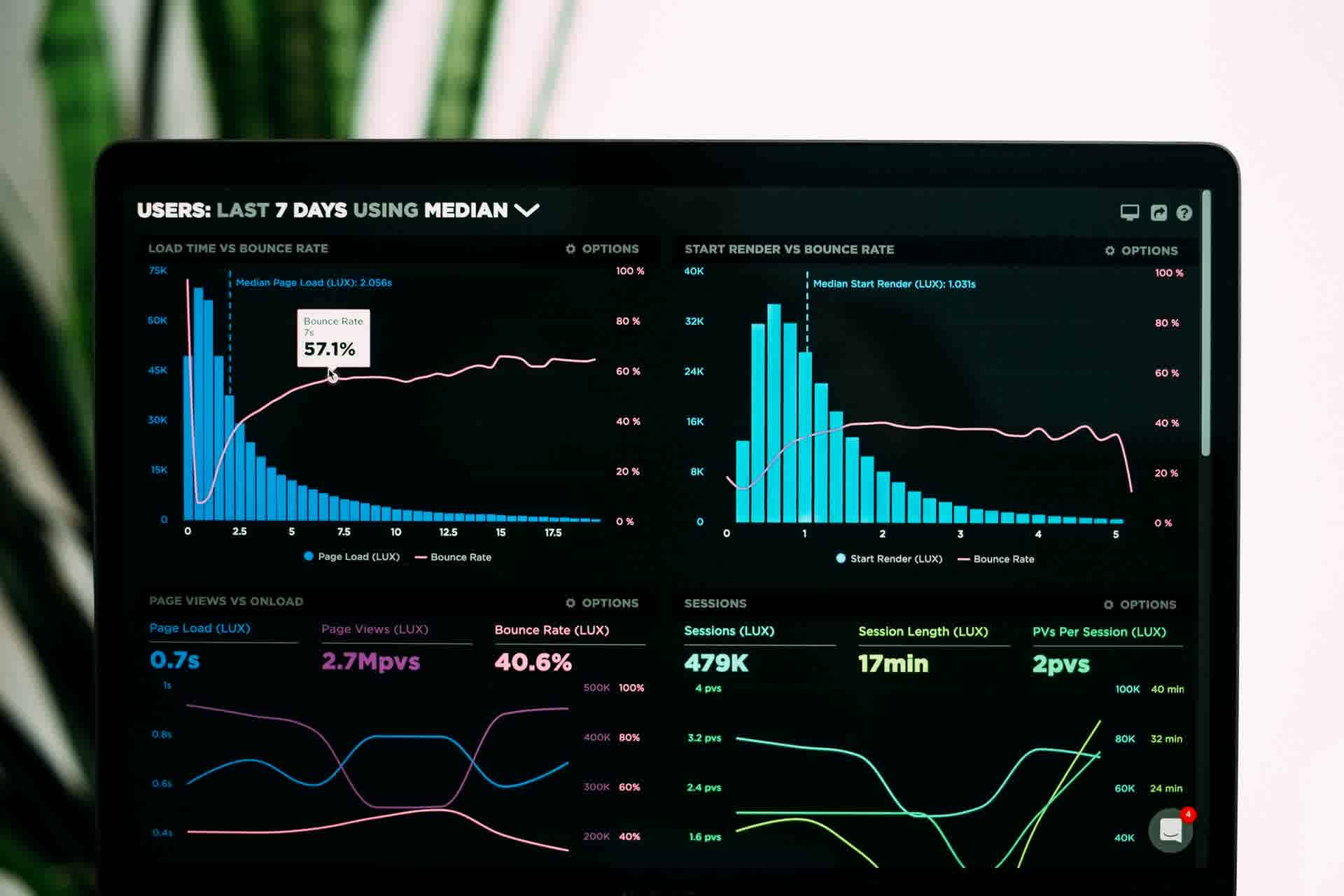
Photo by
Luke Chesser
Often we may need to send some analytics to the server before a user leaves a webpage. So the first thing that comes to your mind would be to use fetch
or XHR
(XML Http Request) API before document unloads i.e before the user leaves.
// when document unloads
// or when user leaves
window.addEventListener("unload", () => {
// send data to /analytics api endpoint in server
fetch('/analytics',{
method: 'post',
body: analyticsData
})
.then(()=> console.log("✅ Success: User analytics send"));
.catch(()=>console.log("🚨 Error: User analytics not send"));
})
But this fetch request is ignored by the browser most of the time even though this is an asynchronous request to improve load times of the next page.
This is where the navigator.sendBeacon()
method comes into the play.
// when document unloads
// or when user leaves
window.addEventListener("unload", () => {
//send data to /analytics api endpoint in server
// using navigator.sendBeacon() method
navigator.sendBeacon("/analytics", analyticsData);
});
The navigator.sendBeacon()
method:
- returns boolean
true
if the browser queues data for transfer andfalse
if not. - requires the API endpoint to send data as the first argument.
- requires the analytics data to be sent to the server as the second argument.
- It is an asynchronous request and doesn't need a response and is guaranteed to run.
- doesn't block the loading of the next page.
- much simpler than
fetch
andXHR
API.