To make the object properties string
type values to string literals
, we can use the as
keyword after the property string
value and then type out the string literal or we can use the as
keyword after accessing the property then typing out the string literal.
TL;DR
METHOD 1
// a simple object
// with "name" property type changed
// from "string" to "John Doe" string literal
const person = {
name: "John Doe" as "John Doe",
age: 23,
};
METHOD 2
// a simple object
const person = {
name: "John Doe",
age: 23,
};
// a simple function call
// with "name" property type changed
// from "string" to "John Doe" string literal
sayName(person.name as "John Doe");
To understand it better, let's say we have an object called person
with two properties called name
and age
with values of John Doe
and 23
respectively like this,
// a simple object
const person = {
name: "John Doe",
age: 23,
};
Now if you hover over the name
property in the person
object it shows the type of the name
property as string
like in the below image,
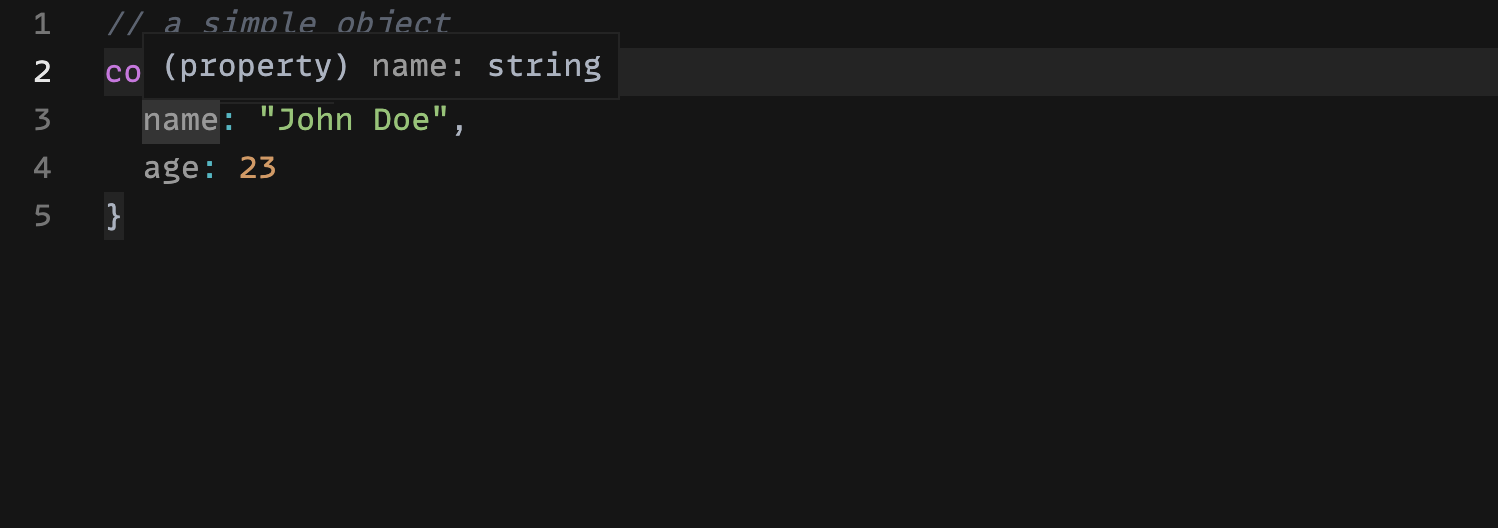
This is ok for most cases. But in some cases, you may need to convert the name
property's type from just string
to a string literal
to pass it to a function call as an argument.
To do that, we can use the as
keyword after the name
property's string value and then type the string literal
we need to use. In our case, we need to change it to John Doe
string literal type. It can be done like this,
// a simple object
// with "name" property type converted
// from just "string" to "John Doe" string literal
const person = {
name: "John Doe" as "John Doe",
age: 23,
};
Now if you hover over the name
property you can see that the type of the name
property is changed from string
to John Doe
string literal which is what we want.
It may look like this,
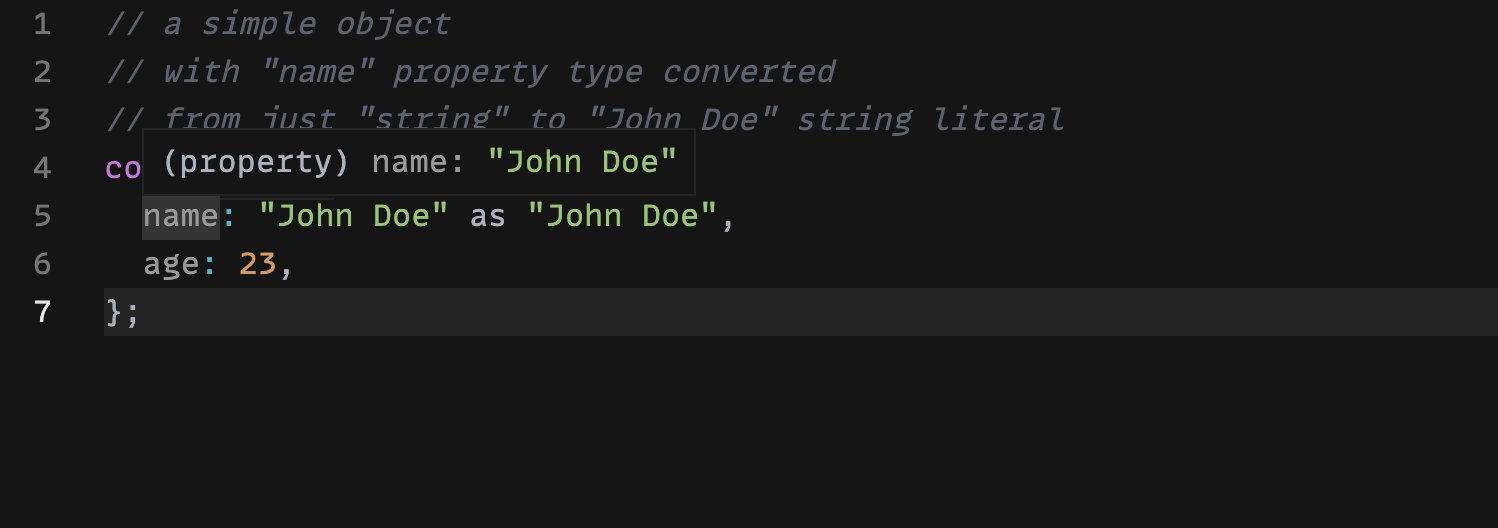
Another way of achieving the same functionality is using the as
keyword and typing the string literal when we are only accessing the property from the object.
For example, let's assume a function called sayName
, and on calling the function let's pass the name
property value like this,
// a simple object
const person = {
name: "John Doe",
age: 23,
};
// a simple function call
sayName(person.name);
Now to change the type of the name
property from string
to "John Doe" string literal
we can use the as
keyword after the person.name
and type the string literal like this,
// a simple object
const person = {
name: "John Doe",
age: 23,
};
// a simple function call
// with "name" property type changed
// from "string" to "John Doe" string literal
sayName(person.name as "John Doe");
This is also a valid way of achieving the same functionality.
See the working of the above codes live in codesandbox
That's all 😃!