To make the object properties boolean
type values to boolean literals
, we can use the as
keyword after the property boolean
value and then type out the boolean literals true
or false
or we can use the as
keyword after accessing the property then typing out the boolean literals true
or false
.
TL;DR
METHOD 1
// a simple object
// with "isAdmin" property type changed
// from "boolean" to "true" boolean literal
const person = {
name: "John Doe",
isAdmin: true as true,
};
METHOD 2
// a simple object
const person2 = {
name: "John Doe",
isAdmin: true,
};
// a simple function call
// with "isAdmin" property type changed
// from "boolean" to true boolean literal
sayIsAdmin(person2.isAdmin as true);
To understand it better, let's say we have an object called person
with two properties called name
and isAdmin
with values of John Doe
and true
respectively like this,
// a simple object
const person = {
name: "John Doe",
isAdmin: true,
};
Now if you hover over the isAdmin
property in the person
object it shows the type of the isAdmin
property as boolean
like in the below image,
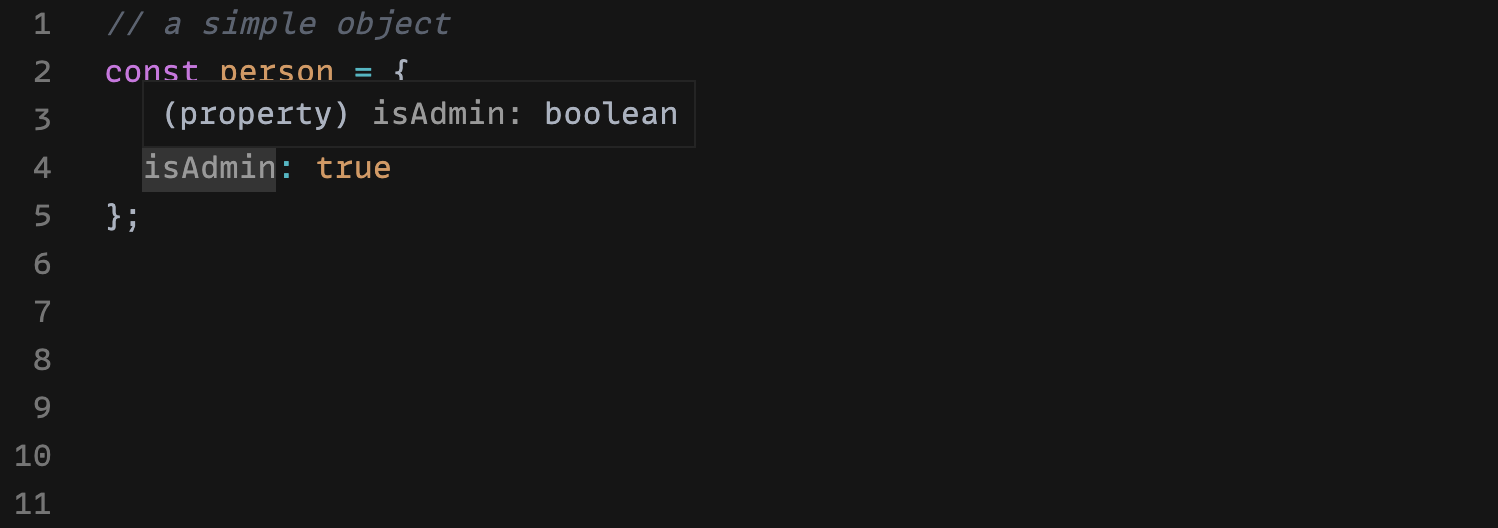
This is ok for most cases. But in some cases, you may need to convert the isAdmin
property's type from just boolean
to a boolean literal
to pass it to a function call as an argument.
To do that, we can use the as
keyword after the isAdmin
property's boolean value and then type the boolean literal
(either true
or false
) we need to use. In our case, let's change it to true
boolean literal type. It can be done like this,
// a simple object
// with "isAdmin" property type converted
// from just "boolean" to "true" boolean literal
const person = {
name: "John Doe",
isAdmin: true as true,
};
Now if you hover over the isAdmin
property you can see that the type of the isAdmin
property is changed from boolean
to true
boolean literal which is what we want.
It may look like this,
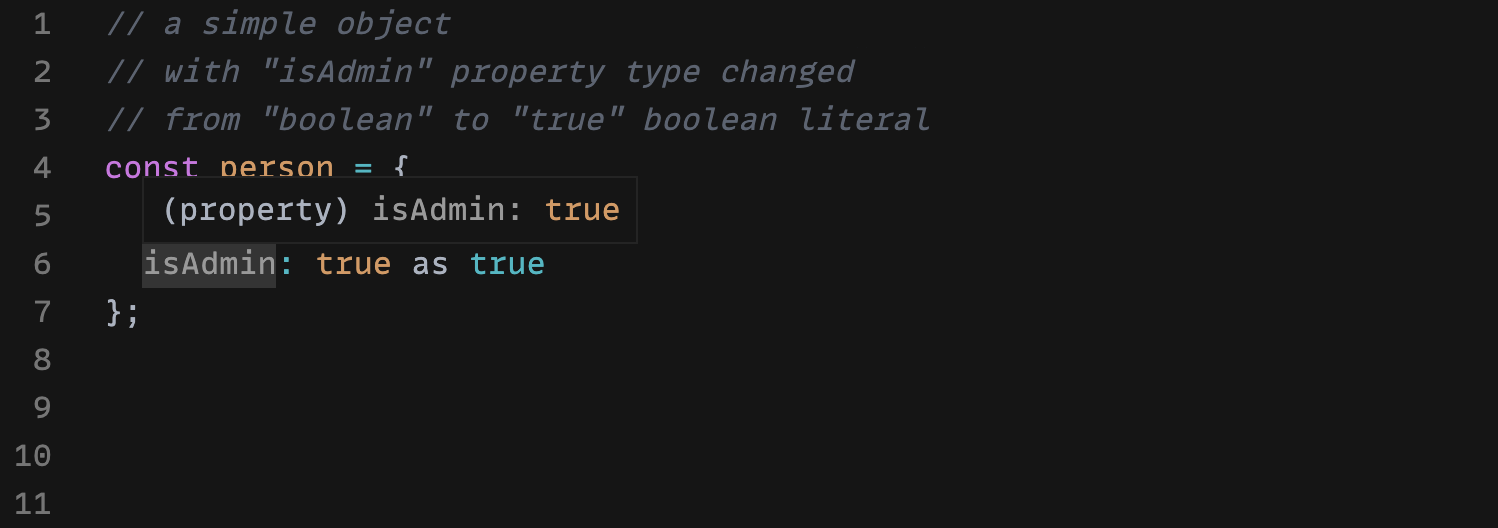
Another way of achieving the same functionality is using the as
keyword and typing the boolean literal when we are only accessing the property from the object.
For example, let's assume a function called sayIsAdmin
, and on calling the function let's pass the isAdmin
property value like this,
// a simple object
const person = {
name: "John Doe",
isAdmin: true,
};
// a simple function call
sayIsAdmin(person.isAdmin);
Now to change the type of the isAdmin
property from boolean
to "true" boolean literal
we can use the as
keyword after the person.isAdmin
and type the boolean literal like this,
// a simple object
const person = {
name: "John Doe",
isAdmin: true,
};
// a simple function call
// with "isAdmin" property type changed
// from "boolean" to "true" boolean literal
sayIsAdmin(person.isAdmin as true);
This is also a valid way of achieving the same functionality.
See the working of the above codes live in codesandbox
That's all 😃!