To load an SVG file from a remote url, you can use the img
HTML tag and then provide the SVG remote url as the value to the src
attribute.
TL;DR
<html>
<body>
<!-- Using `img` HTML tag to load SVG file from a remote url -->
<img
src="https://upload.wikimedia.org/wikipedia/commons/thumb/1/12/Black_Paw.svg/852px-Black_Paw.svg.png"
decoding="async"
loading="lazy"
width="200"
height="200"
/>
</body>
</html>
For example, let's say we have an SVG hosted at this url.
We aim to load this SVG url and render this SVG on the webpage. To do that, we can use the img
HTMl tag and then use the src
attribute and set its value as the SVG remote url which is https://upload.wikimedia.org/wikipedia/commons/thumb/1/12/Black_Paw.svg/852px-Black_Paw.svg.png
.
It can be done like this,
<html>
<body>
<!-- Using `img` HTML tag to load SVG file from a remote url -->
<img
src="https://upload.wikimedia.org/wikipedia/commons/thumb/1/12/Black_Paw.svg/852px-Black_Paw.svg.png"
/>
</body>
</html>
The webpage now looks like this,
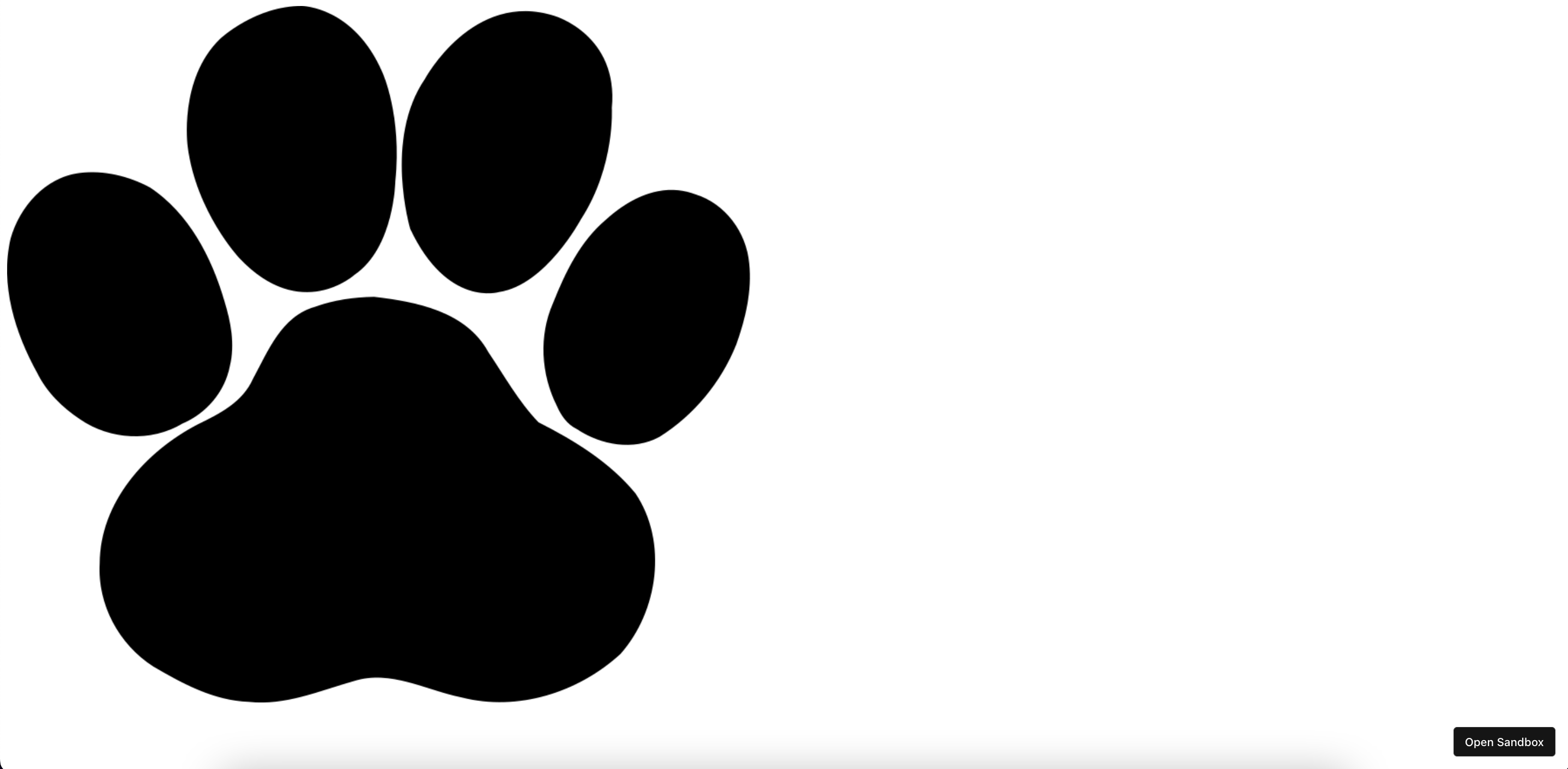
Now to improve the webpage performance while loading the SVG file, we can define more attributes on the img
tag like decoding="async"
, and loading="lazy"
. We can also set its width and height using the attributes width
and height
and set both of its values to 200
.
The html now looks like this,
<html>
<body>
<!-- Using `img` HTML tag to load SVG file from a remote url -->
<img
src="https://upload.wikimedia.org/wikipedia/commons/thumb/1/12/Black_Paw.svg/852px-Black_Paw.svg.png"
decoding="async"
loading="lazy"
width="200"
height="200"
/>
</body>
</html>
The webpage is now more performant and also the SVG is resized to the width and height defined in the attributes.
The webpage now looks like this,
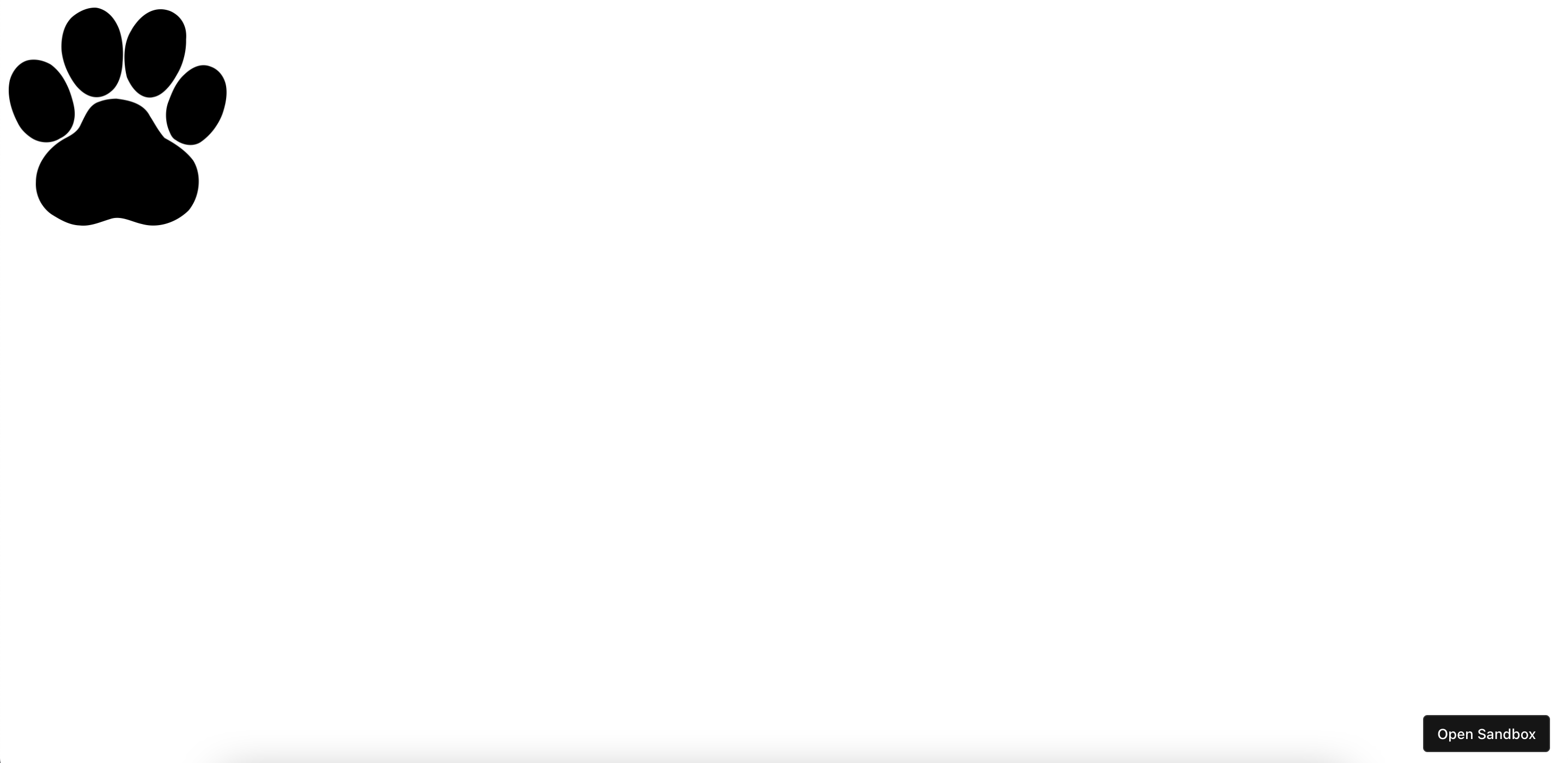
We have successfully loaded an SVG file from a remote url. Yay 🥳!
See the above code live in the codesandbox.
That's all 😃.