To get or access the properties and methods of the figure
HTML element tag without having errors or red squiggly lines in TypeScript, you have to assert the type for the figure
HTML element tag using the HTMLElement
interface in TypeScript.
The errors or warnings are shown by the TypeScript compiler since the compiler doesn't know more information on the figure
HTML element tag ahead of time. But we know that we are referencing the figure
HTML tag and thus we can tell the compiler to use the HTMLElement
interface which contains the declarations for properties and methods.
TL;DR
// get reference to the first 'figure'
// HTML element tag in the document
// with type assertion using the HTMLElement interface
const figureTag = document.querySelector("figure") as HTMLElement;
// no errors or red squiggly lines will be
// shown while accessing properties or methods
// since we have asserted the type
// for the 'figure' HTML element tag 😍
const id = figureTag.id;
To understand it better let's say we are selecting the first figure
HTML element tag using the document.querySelector()
method like this,
// get reference to the first 'figure'
// HTML element tag in the document
const figureTag = document.querySelector("figure");
Now let's try to get the value of a property called id
which is used in the figure
HTML element tag as an attribute.
It can be done like this,
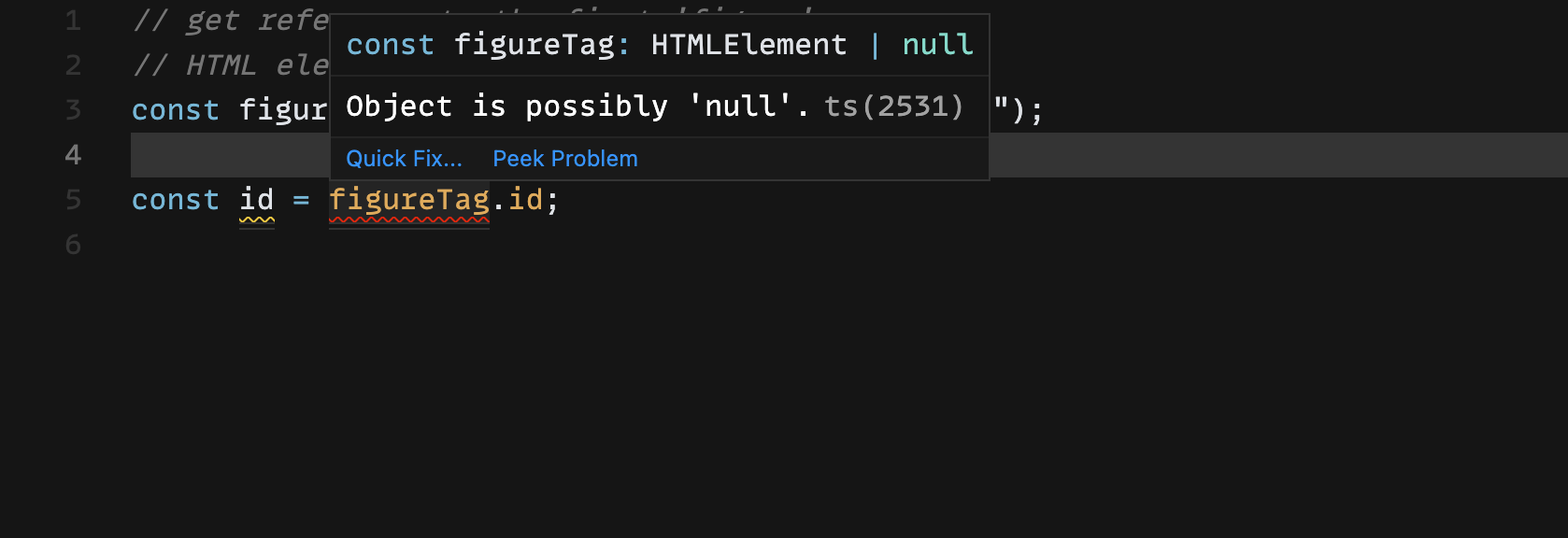
As you can see that the TypeScript compiler is showing a red squiggly line below the figureTag
object. If you hover over the figureTag
you can see an error saying Object is possibly 'null'
. This is because TypeScript is not sure whether the figureTag
object will have properties and methods ahead of time.
Now to avoid this error we can assert the type for the figure
HTML element tag using the HTMLElement
interface in TypeScript.
To do Type assertion we can use the as
keyword after the document.querySelector("figure")
method followed by writing the interface name HTMLElement
.
It can be done like this,
// get reference to the first 'figure'
// HTML element tag in the document
// with type assertion using the HTMLElement interface
const figureTag = document.querySelector("figure") as HTMLElement;
// no errors or red squiggly lines will be
// shown while accessing properties or methods
// since we have asserted the type
// for the 'figure' HTML element tag 😍
const id = figureTag.id;
Now if hover over the id
property in the figureTag
object TypeScript shows more information about the property itself which is again cool and increases developer productivity and clarity while coding.
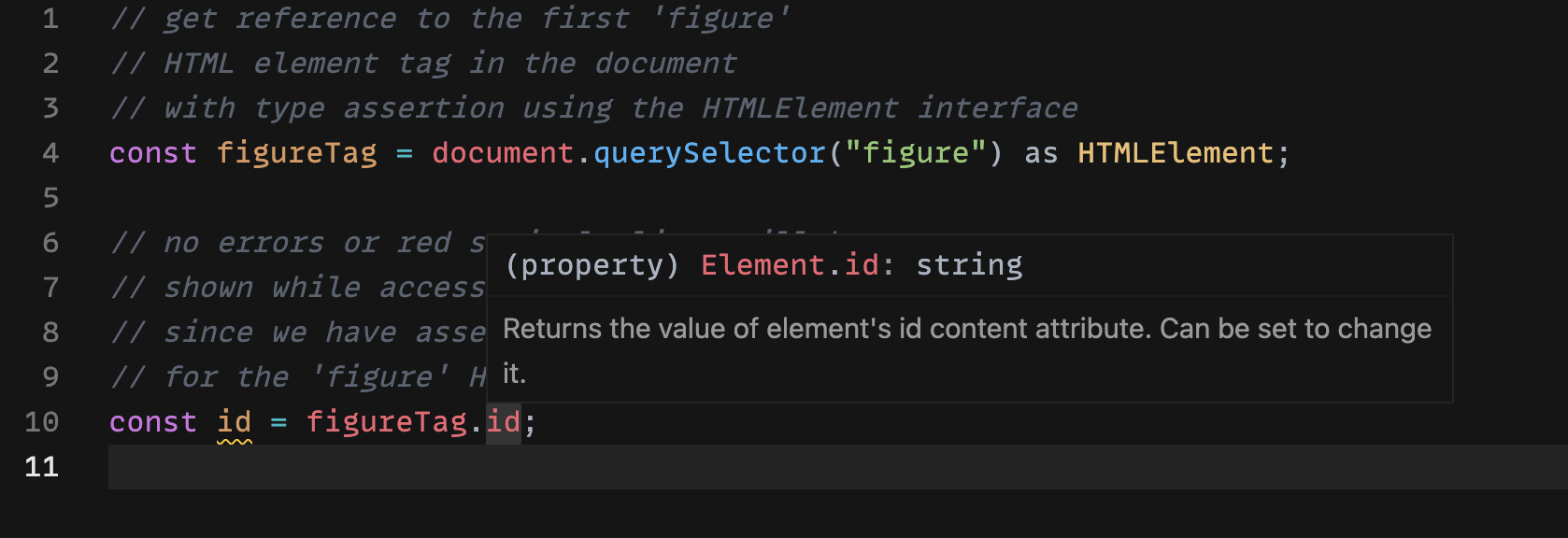
See the above code live in codesandbox.
That's all 😃!