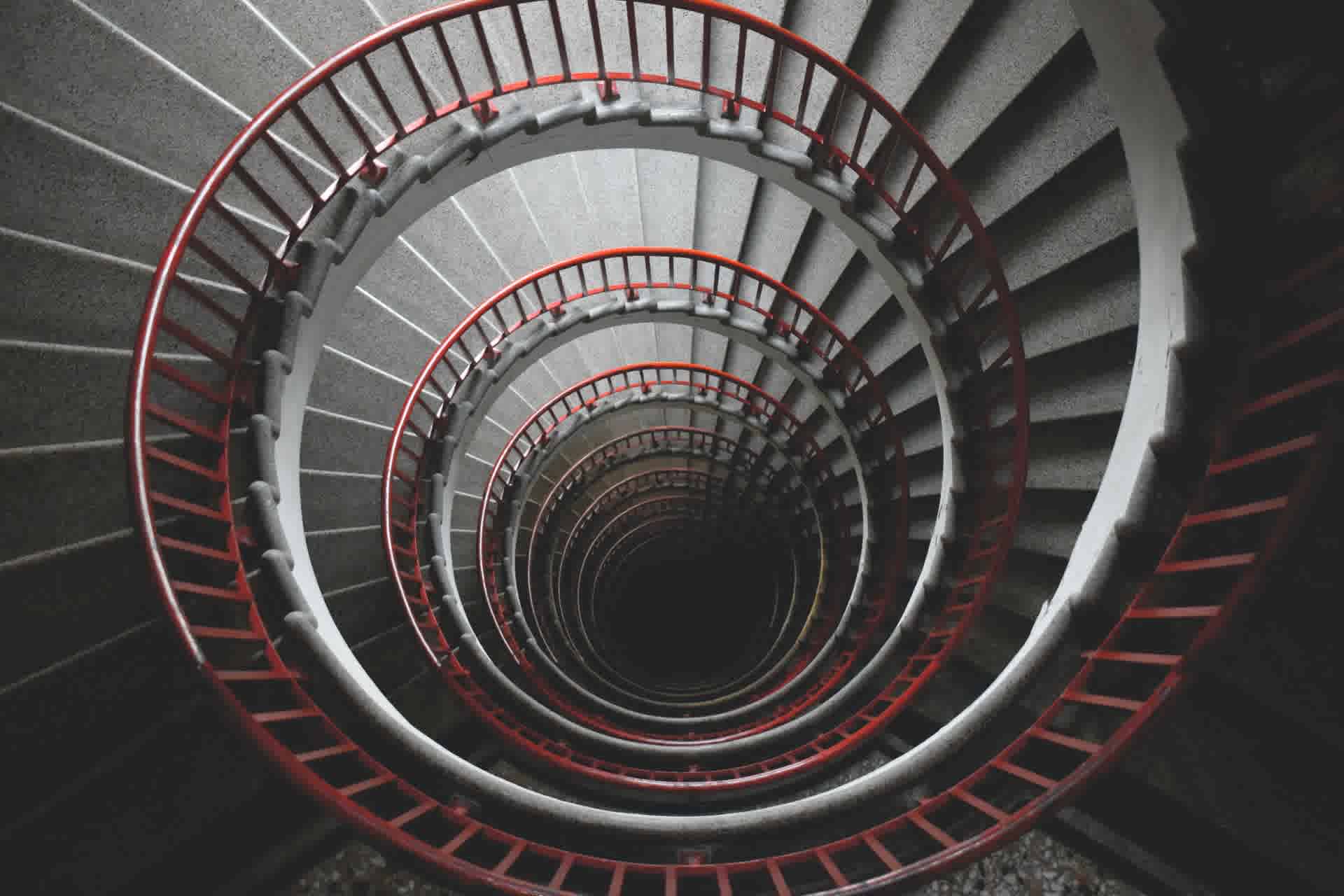
Photo by
Tine Ivanič
for...in
statement
The for...in
statement is used to loop over object enumerable properties.
Let's consider an object called john
with name
and age
fields
// object
const john = {
name: "John Doe",
age: 23,
};
// loop over object
for (let key in john) {
console.log(key); // Result: "name", "age"
}
If we loop over an array it will loop over the index value since the arrays are objects in their underlying nature.
// array
const john = ["John Doe", 23];
// loop over array
for (let key in john) {
console.log(key); // Result: "0" , "1"
}
for...of
statement
These are a little different from the for...in
statement.
The for...of
statement is used to loop over the values of iterable objects only which includes Arrays, Maps, etc.
The for...of
statement gives out the values instead of indexes.
If we try to loop over objects it will return an error.
Let's try to loop over an object using the for...of
statement.
// object
const john = {
name: "John Doe",
age: 23,
};
// loop over object
for (let key of john) {
console.log(key); // Error
}
Now let's loop over an array
// array
const john = ["John Doe", 23];
// loop over array values
for (let value of john) {
console.log(value); // Result: "John Doe" , 23
}