To convert an entire object to a read-only or a constant object, you can use the as
keyword after the object initialization followed by the keyword const
in TypeScript.
TL;DR
// using "as" keyword after the person
// object initialisation followed by the keyword "const"
// to make the object readonly or a constant object
const person = {
name: "John Doe",
age: 23,
} as const;
// change the "name" property value
person.name = "Hello"; // ❌ not allowed. Cannot assign to 'name' because it is a read-only property.
For example, let' say we have an object called person
with 2 properties called name
and age
having values of John Doe
and 23
respectively like this,
// a simple object
const person = {
name: "John Doe",
age: 23,
};
Now if hover over the person
object variable, you can see that the TypeScript assumed the types of the name
and age
properties to be string
and number
like in the below image,
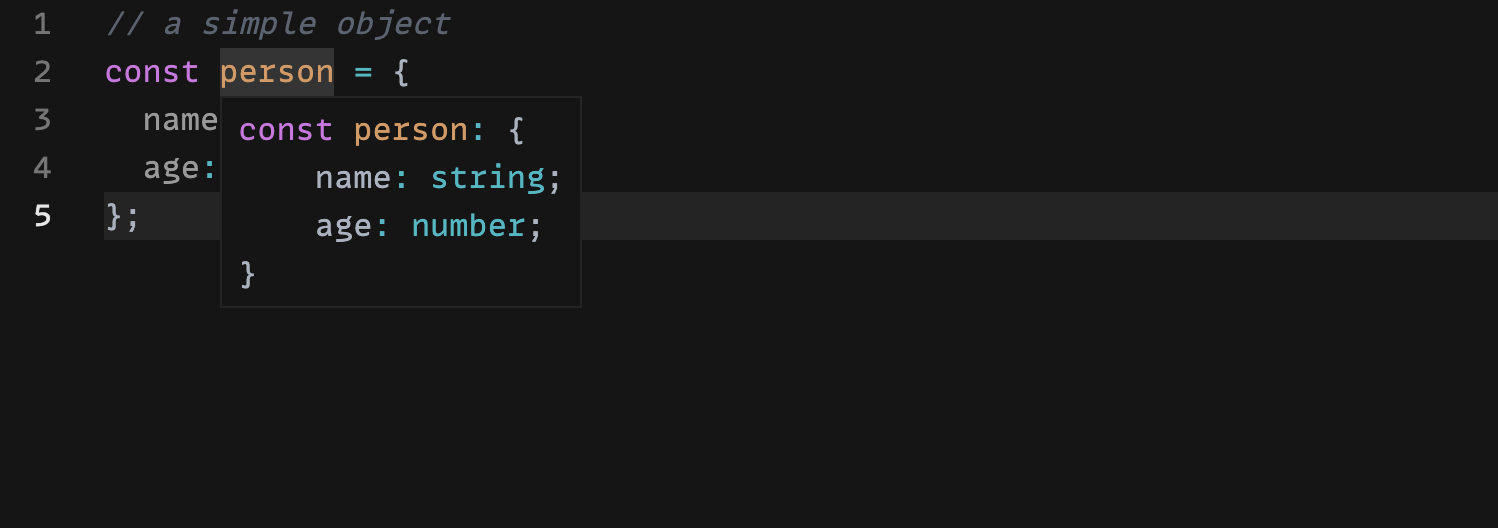
This is fine.
But in some cases, we may need to convert an entire object into a readonly
or a constant
object to avoid changing values of the properties in the object for security reasons.
To do that we can write the as
keyword after the person
object initialization followed by the keyword const
like this,
// using "as" keyword after the person
// object initialization followed by the keyword "const"
// to make the object readonly or a constant object
const person = {
name: "John Doe",
age: 23,
} as const;
If we hover over the person
object now we can see that all the properties inside the person
object have the word readonly
shown to the left of each property which is telling us that all the properties can now be read but not changed.
It may look like this,
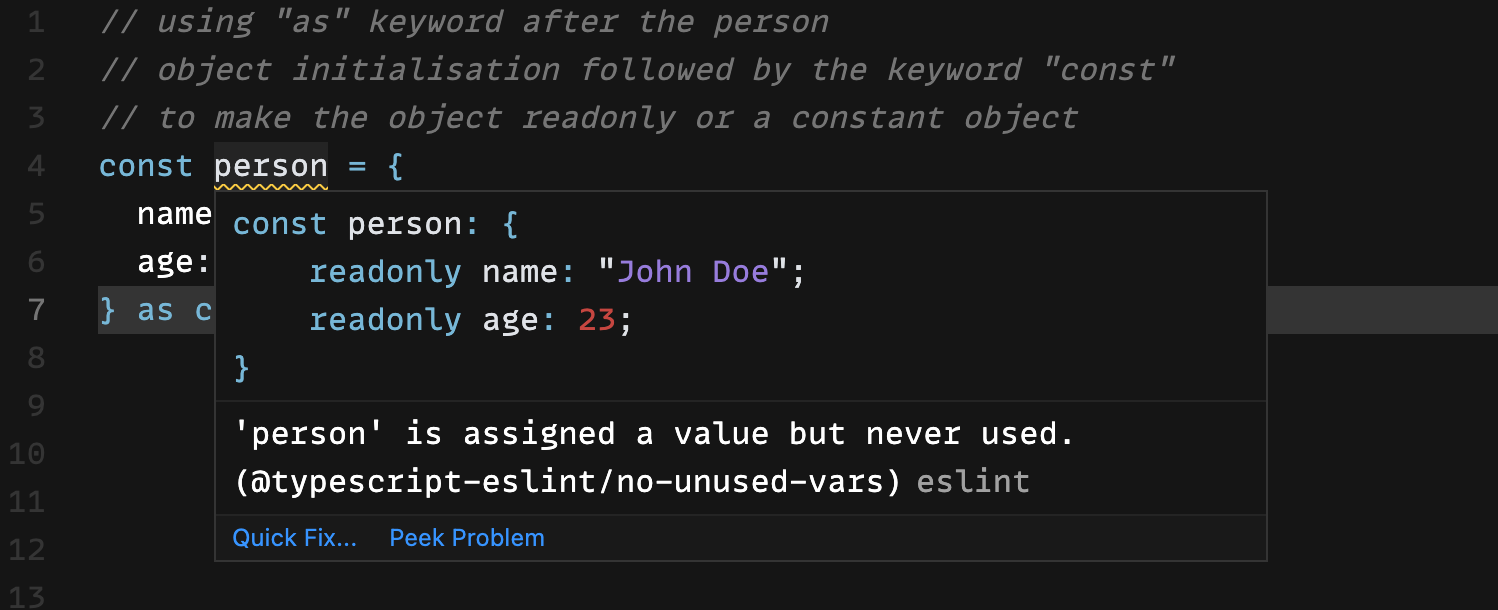
Now let's try to change the value of the name
property from the person
object like this,
// using "as" keyword after the person
// object initialization followed by the keyword "const"
// to make the object readonly or a constant object
const person = {
name: "John Doe",
age: 23,
} as const;
// change the "name" property value
person.name = "Hello"; // ❌ not allowed. Cannot assign to 'name' because it is a read-only property.
As you can see from the above code that the TypeScript compiler will not allow changing the value of the name
property and shows an error saying Cannot assign to 'name' because it is a read-only property.
which is exactly what we want to happen.
See the above code live in codesandbox.
That's all 😃!