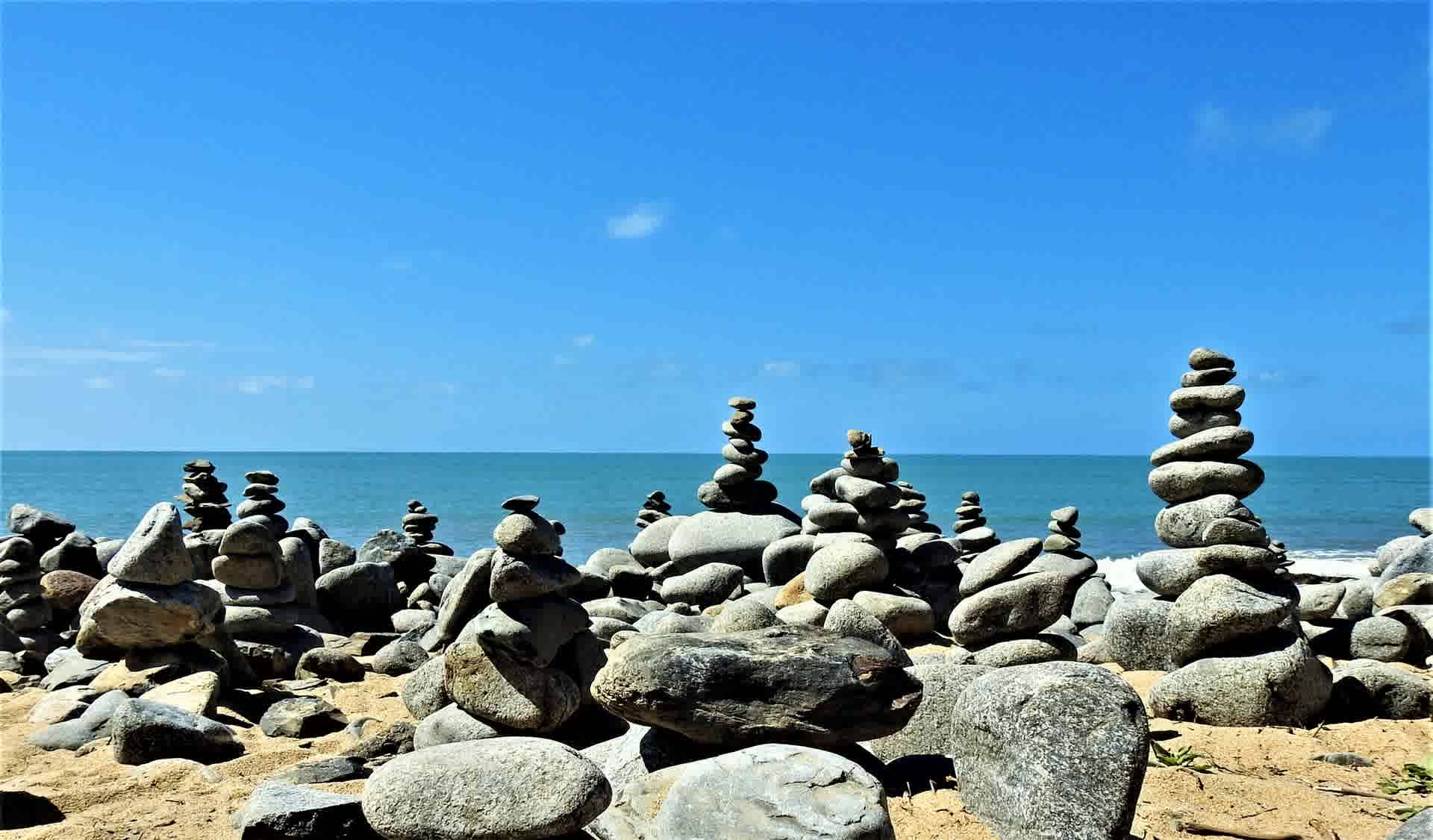
Photo by
Laya Clode
In many cases, we have null
or undefined
values in our variables. We can conditionally show other relevant value using the new ??
(Nullish coalescing) operator instead of just showing null
or undefined
values.
Consider these variables name
with the value of null
and age
with the value of 23
.
// name and age variables
let name = null;
let age = 23;
Now if we try to view the values using the console.log() method it would show null
and 23
in the console.
// name and age variables
let name = null;
let age = 23;
console.log(name, age);
But we don't want an unpredictable behavior like that.
Let's now remove that unpredictable behavior using the ??
operator.
// name and age variables
let name = null;
let age = 23;
// using ?? operator
console.log(name ?? "Anonymous", age ?? "Age not defined");
Now if we try to view the variables we can see that when showing the name
variable it shows Anonymous
and for the age
variable it shows the number 23.
This is what the ??
operator does.
It checks if a variable has null
or undefined
values and if it does contain those values it doesn't take that value and gives it what comes after the ??
operator. In our case Anonymous
value.
Now if you try to change the age
value to undefined
.
it will show,
Anonymous
Age not defined