To avoid the width
or height
getting increased or decreased when using CSS properties like margin
, padding
, etc, we can use the CSS property called box-sizing
and set its value to border-box
on the element in CSS.
TL;DR
HTML
<!-- div HTML tag -->
<div>Hello World!</div>
CSS
.myDiv {
height: 100px;
width: 200px;
background-color: red;
padding: 20px;
box-sizing: border-box; /* add this */
}
For example, first let's make a div
HTML element tag with content Hello World!
inside it like this,
<!-- div HTML tag -->
<div>Hello World!</div>
Now let's add a class name called myDiv
so that we can reference this div
in CSS. It can be done like this,
<!-- div HTML tag -->
<div class="myDiv">Hello World!</div>
Now inside our CSS we can use the myDiv
class and set the div
HTML element tag's height to 100px
, its width to 200px
and a background color of red
. It can be done like this,
.myDiv {
height: 100px;
width: 200px;
background-color: red;
}
Till here we are ok. We have a well-shaped box with a height of 100px
and a width of 200px.
Now to understand the problem a little better, let's also add a padding
of 20px
to the myDiv
like this,
.myDiv {
height: 100px;
width: 200px;
background-color: red;
padding: 20px;
}
Now the div
element's height and width are also increased because the padding added 10px
to all the four sides of the div
element, so now the height of the div
becomes 140px
and the width becomes 240px
which may make styling sometimes a bit tricky and unpredictable.
I have added a small gif to show the problem. This is what will happen to the div
element if the padding is added and not added.
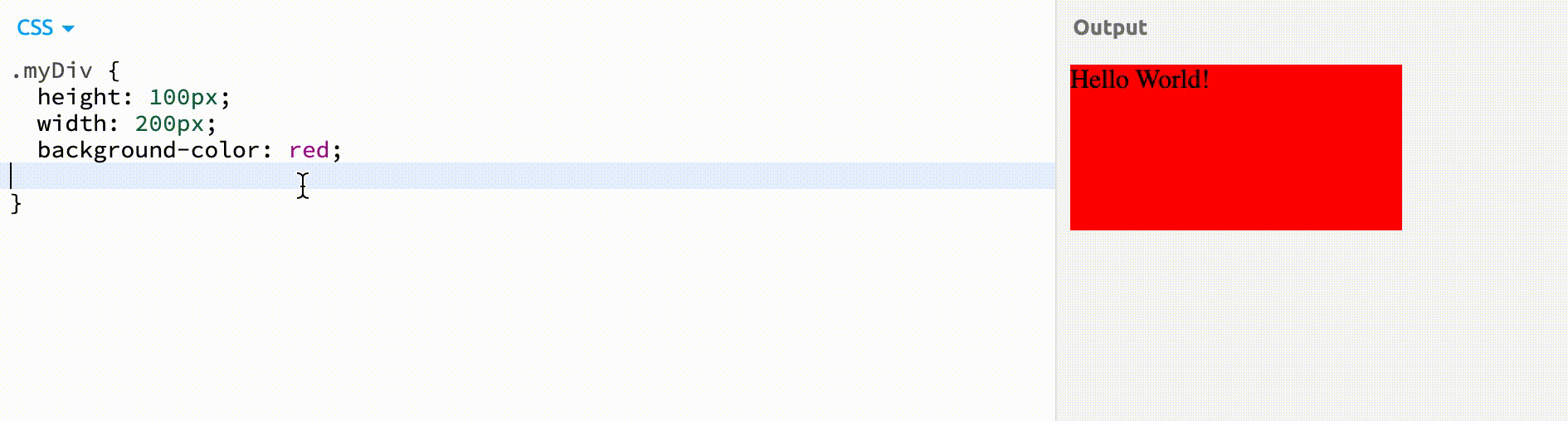
So why does this happen?
This is because browsers by default add all the padding
, margins
, etc to the overall height
and width
of the element, in our case it is the div
HTML element.
By default, the box-sizing
CSS property of the element will be set to content-box
which causes this unpredictable behavior.
To avoid this issue we can use the box-sizing
property and set is value to border-box
like this,
.myDiv {
height: 100px;
width: 200px;
background-color: red;
padding: 20px;
box-sizing: border-box;
}
Now we will have something where the height
and width
of the overall div
element are not increased but restricted with the defined height and width of the box.
It will look like this,
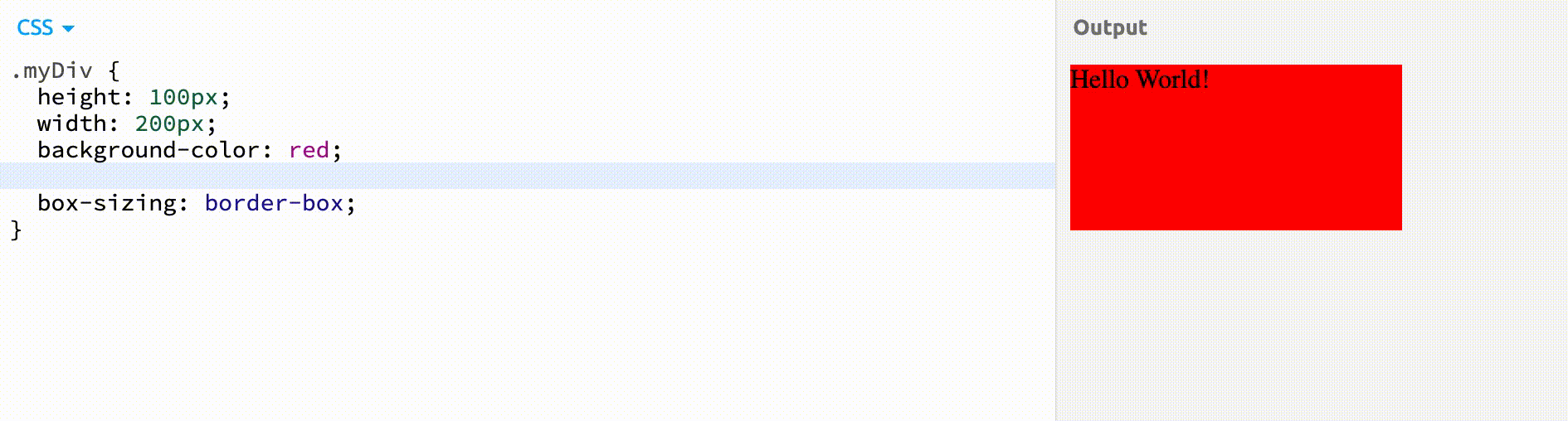
Which is the result we need! Yay 🎊!
See the above code live in JSBin
That's all! 😃